Fix Material 3 Scrollable `TabBar` (#125974)
fix https://github.com/flutter/flutter/issues/117722
### Description
1. Fix the divider doesn't stretch to take all the available width in the scrollable tab bar in M3
2. Add `dividerHeight` property.
3. Update the default tab alignment for the scrollable tab bar to match the specs (this is backward compatible for M2 with the new `tabAlignment` property).
### Bug (default tab alignment)
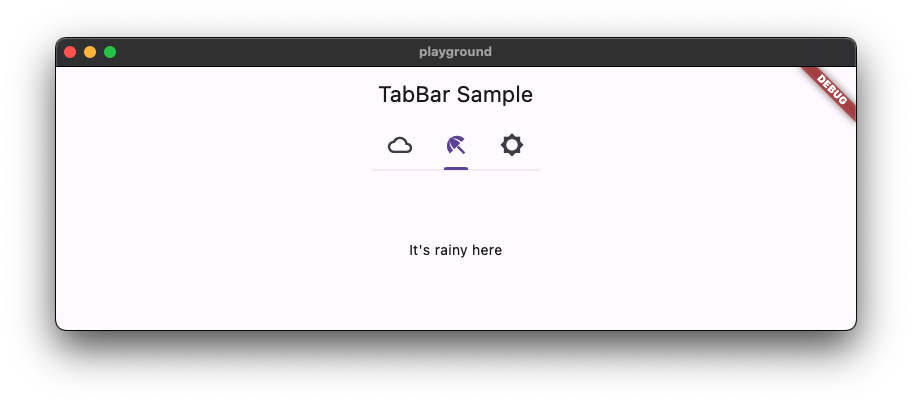
### Fix (default tab alignment)
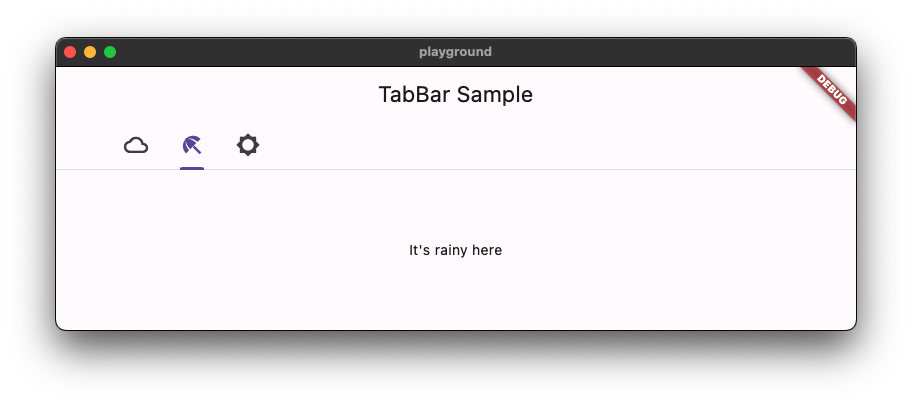
### Code sample
<details>
<summary>code sample</summary>
```dart
import 'package:flutter/material.dart';
/// Flutter code sample for [TabBar].
void main() => runApp(const TabBarApp());
class TabBarApp extends StatelessWidget {
const TabBarApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
theme: ThemeData(
// tabBarTheme: const TabBarTheme(tabAlignment: TabAlignment.start),
useMaterial3: true,
),
home: const TabBarExample(),
);
}
}
class TabBarExample extends StatefulWidget {
const TabBarExample({super.key});
@override
State<TabBarExample> createState() => _TabBarExampleState();
}
class _TabBarExampleState extends State<TabBarExample> {
bool rtl = false;
@override
Widget build(BuildContext context) {
return DefaultTabController(
initialIndex: 1,
length: 3,
child: Directionality(
textDirection: rtl ? TextDirection.rtl : TextDirection.ltr,
child: Scaffold(
appBar: AppBar(
title: const Text('TabBar Sample'),
),
body: const Column(
children: <Widget>[
Text('Scrollable-TabAlignment.start'),
TabBar(
isScrollable: true,
tabAlignment: TabAlignment.start,
tabs: <Widget>[
Tab(
icon: Icon(Icons.cloud_outlined),
),
Tab(
icon: Icon(Icons.beach_access_sharp),
),
Tab(
icon: Icon(Icons.brightness_5_sharp),
),
],
),
Text('Scrollable-TabAlignment.startOffset'),
TabBar(
isScrollable: true,
tabAlignment: TabAlignment.startOffset,
tabs: <Widget>[
Tab(
icon: Icon(Icons.cloud_outlined),
),
Tab(
icon: Icon(Icons.beach_access_sharp),
),
Tab(
icon: Icon(Icons.brightness_5_sharp),
),
],
),
Text('Scrollable-TabAlignment.center'),
TabBar(
isScrollable: true,
tabAlignment: TabAlignment.center,
tabs: <Widget>[
Tab(
icon: Icon(Icons.cloud_outlined),
),
Tab(
icon: Icon(Icons.beach_access_sharp),
),
Tab(
icon: Icon(Icons.brightness_5_sharp),
),
],
),
Spacer(),
Text('Non-scrollable-TabAlignment.fill'),
TabBar(
tabAlignment: TabAlignment.fill,
tabs: <Widget>[
Tab(
icon: Icon(Icons.cloud_outlined),
),
Tab(
icon: Icon(Icons.beach_access_sharp),
),
Tab(
icon: Icon(Icons.brightness_5_sharp),
),
],
),
Text('Non-scrollable-TabAlignment.center'),
TabBar(
tabAlignment: TabAlignment.center,
tabs: <Widget>[
Tab(
icon: Icon(Icons.cloud_outlined),
),
Tab(
icon: Icon(Icons.beach_access_sharp),
),
Tab(
icon: Icon(Icons.brightness_5_sharp),
),
],
),
Spacer(),
],
),
floatingActionButton: FloatingActionButton.extended(
onPressed: () {
setState(() {
rtl = !rtl;
});
},
label: const Text('Switch Direction'),
icon: const Icon(Icons.swap_horiz),
),
),
),
);
}
}
```
</details>

https://dart.googlesource.com/external/github.com/flutter/flutter/+/32fde139bc9cd03090643985e146e6ad8be75663
1 file changed